当尝试在C#控制台应用程序中通过Azure CLI运行命令时,遇到了一个异常。虽然编译过程中没有错误,但在执行项目时出现了错误。
以下是遇到的错误截图:
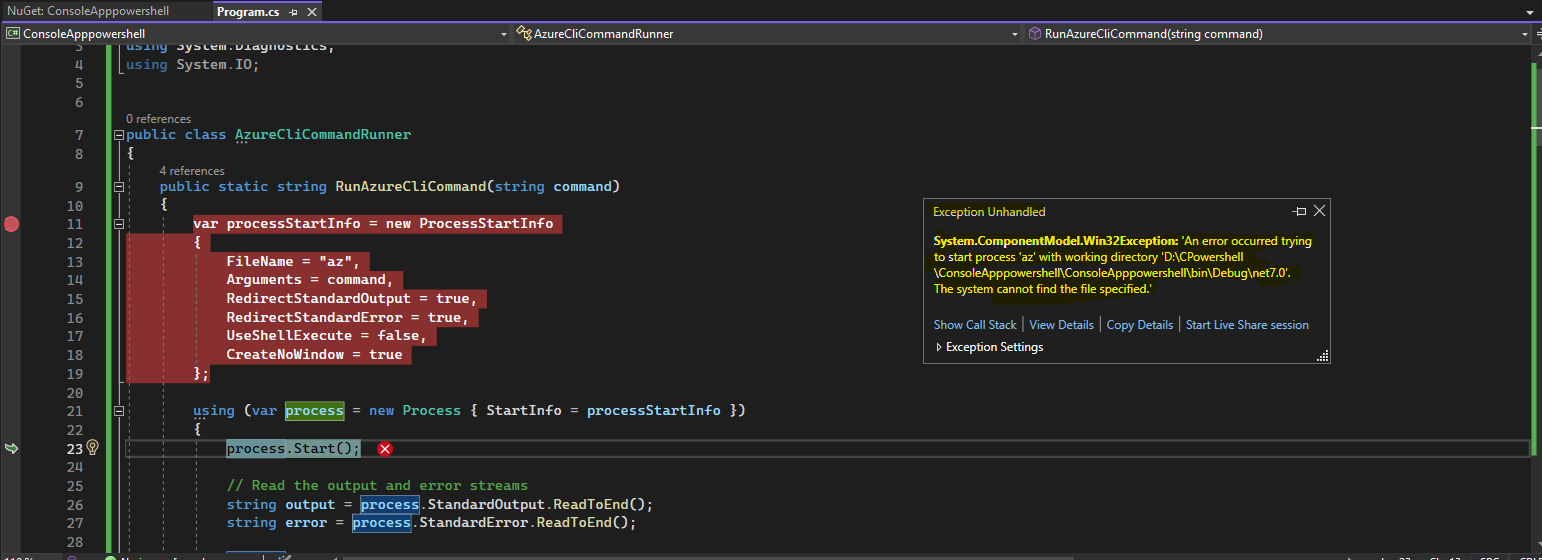
下面是我要运行的C#代码:
using System;
using System.Diagnostics;
using System.IO;
public class AzureCliCommandRunner
{
public static string RunAzureCliCommand(string command)
{
var processStartInfo = new ProcessStartInfo
{
FileName = "az",
Arguments = command,
RedirectStandardOutput = true,
RedirectStandardError = true,
UseShellExecute = false,
CreateNoWindow = true
};
using (var process = new Process { StartInfo = processStartInfo })
{
process.Start();
// 读取输出和错误流
string output = process.StandardOutput.ReadToEnd();
string error = process.StandardError.ReadToEnd();
process.WaitForExit();
if (process.ExitCode == 0)
{
return output;
}
else
{
// 处理错误
throw new InvalidOperationException($"命令执行失败。错误:{error}");
}
}
}
public static void UpdateAppConfigFilter(string endpoint, string featureName)
{
// 运行Azure CLI命令以更新AppConfig过滤器
string command1 = $"appconfig feature filter show --connection-string {endpoint} --feature {featureName} --filter-name Microsoft.Targeting";
string jsondata = RunAzureCliCommand(command1);
string command2 = $"echo '{jsondata}' | jq -r '.[].parameters.Audience'";
string audienceSection = RunAzureCliCommand(command2).Trim();
string new_user = "newuser";
string command3 = $"echo '{audienceSection}' | jq --arg new_user '{new_user}' '.Users += [\"$new_user\"]'";
string file_content = RunAzureCliCommand(command3).Trim();
string command4 = $"appconfig feature filter update --connection-string {endpoint} --feature {featureName} --filter-name Microsoft.Targeting --filter-parameters Audience='{file_content}' --yes";
RunAzureCliCommand(command4);
}
public static void Main()
{
// 将 <<EndPoint>> 和 <<FeatureName>> 替换为实际值
string endpoint = "<<EndPoint>>";
string featureName = "<<FeatureName>>";
UpdateAppConfigFilter(endpoint, featureName);
}
}
这段C#代码定义了一个名为AzureCliCommandRunner
的类,该类包含一个用于运行Azure CLI命令的方法RunAzureCliCommand
以及一个用于更新AppConfig过滤器的方法UpdateAppConfigFilter
。在Main
方法中调用UpdateAppConfigFilter
并传入实际的端点(endpoint)和特性名称(featureName)。错误提示表明在执行Azure CLI命令时遇到了问题,需要查看错误信息以确定具体原因,并相应地进行调试。